SpringBoot中使用Cookie實(shí)現(xiàn)記住登錄的示例代碼
最近在做項(xiàng)目,甲方提出每次登錄都要輸入密碼,會很麻煩,要求實(shí)現(xiàn)一個記住登錄狀態(tài)的功能,于是便使用 Cookie 實(shí)現(xiàn)該功能
一、Cookie 簡介
Cookie,一種儲存在用戶本地終端上的數(shù)據(jù),有時也用其復(fù)數(shù)形式 Cookies。類型為“小型文本文件”,是某些網(wǎng)站為了辨別用戶身份,進(jìn)行 Session 跟蹤而儲存在用戶本地終端上的數(shù)據(jù)(通常經(jīng)過加密),由用戶客戶端計(jì)算機(jī)暫時或永久保存的信息。
其實(shí) Cookie 就是一個鍵和一個值構(gòu)成的,隨著服務(wù)器端的響應(yīng)發(fā)送給客戶端瀏覽器。然后客戶端瀏覽器會把 Cookie 保存起來,當(dāng)下一次再訪問服務(wù)器時把 Cookie 再發(fā)送給服務(wù)器。
1、Cookie 是 HTTP 協(xié)議的規(guī)范之一,它是服務(wù)器和客戶端之間傳輸?shù)男?shù)據(jù)2、首先由服務(wù)器通過響應(yīng)頭把 Cookie 傳輸給客戶端,客戶端會將 Cookie 保存起來3、當(dāng)客戶端再次請求同一服務(wù)器時,客戶端會在請求頭中添加該服務(wù)器保存的 Cookie,發(fā)送給服務(wù)器4、Cookie 就是服務(wù)器保存在客戶端的數(shù)據(jù)5、Cookie 就是一個鍵值對
二、Cookie 使用
1、創(chuàng)建 Cookie
// Cookie 為鍵值對數(shù)據(jù)格式Cookie cookie_username = new Cookie('cookie_username', username);
2、設(shè)置 Cookie 持久時間
// 即:過期時間,單位是:秒(s)cookie_username.setMaxAge(30 * 24 * 60 * 60);
3、設(shè)置 Cookie 共享路徑
// 表示當(dāng)前項(xiàng)目下都攜帶這個cookiecookie_username.setPath(request.getContextPath());
4、向客戶端發(fā)送 Cookie
// 使用 HttpServletResponse 對象向客戶端發(fā)送 Cookieresponse.addCookie(cookie_username);
5、銷毀 Cookie
// 根據(jù) key 將 value 置空Cookie cookie_username = new Cookie('cookie_username', '');// 設(shè)置持久時間為0cookie_username.setMaxAge(0);// 設(shè)置共享路徑cookie_username.setPath(request.getContextPath());// 向客戶端發(fā)送 Cookieresponse.addCookie(cookie_username);
三、進(jìn)入正題
上面我們已經(jīng)了解了 Cookie 是什么,并且知道了 Cookie 的創(chuàng)建以及銷毀的方法,下面,我們就使用 Cookie 實(shí)現(xiàn)記住登錄狀態(tài)的功能,整個項(xiàng)目基于 SpringBoot 實(shí)現(xiàn)
1、注冊攔截器
/*** 注冊攔截器*/@Configurationpublic class WebConfigurer implements WebMvcConfigurer { @Autowired private LoginInterceptor loginHandlerInterceptor; @Override public void addInterceptors(InterceptorRegistry registry) { InterceptorRegistration ir = registry.addInterceptor(loginHandlerInterceptor); // 攔截路徑 ir.addPathPatterns('/*'); // 不攔截路徑 List<String> irs = new ArrayList<String>(); irs.add('/api/*'); irs.add('/wechat/*'); irs.add('/oauth'); ir.excludePathPatterns(irs); }}
我們攔截了所有的請求路徑,放開了 api、wechat 等請求路徑
這里可能會有一個疑問,為什么不放開請求登錄界面的 api 請求路徑呢,原因是我們攔截登錄請求,當(dāng)我們請求登錄界面時,我們已經(jīng)登錄過,那么我們就無需進(jìn)入登錄界面,直接到主界面
我們使用了自定義的一個登錄攔截:LoginInterceptor,在第二步我們會詳細(xì)講解其中的實(shí)現(xiàn)原理
2、登錄攔截
/*** 未登錄攔截器*/@Componentpublic class LoginInterceptor implements HandlerInterceptor { @Autowired private LoginDao dao; @Override public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception { // 獲得cookie Cookie[] cookies = request.getCookies(); // 沒有cookie信息,則重定向到登錄界面 if (null == cookies) { response.sendRedirect(request.getContextPath() + '/login'); return false; } // 定義cookie_username,用戶的一些登錄信息,例如:用戶名,密碼等 String cookie_username = null; // 獲取cookie里面的一些用戶信息 for (Cookie item : cookies) { if ('cookie_username'.equals(item.getName())) {cookie_username = item.getValue();break; } } // 如果cookie里面沒有包含用戶的一些登錄信息,則重定向到登錄界面 if (StringUtils.isEmpty(cookie_username)) { response.sendRedirect(request.getContextPath() + '/login'); return false; } // 獲取HttpSession對象 HttpSession session = request.getSession(); // 獲取我們登錄后存在session中的用戶信息,如果為空,表示session已經(jīng)過期 Object obj = session.getAttribute(Const.SYSTEM_USER_SESSION); if (null == obj) {// 根據(jù)用戶登錄賬號獲取數(shù)據(jù)庫中的用戶信息 UserInfo dbUser = dao.getUserInfoByAccount(cookie_username); // 將用戶保存到session中 session.setAttribute(Const.SYSTEM_USER_SESSION, dbUser); } // 已經(jīng)登錄 return true; }}
3、登錄請求
控制層
/** * 執(zhí)行登錄 */ @PostMapping('login') @ResponseBody public String login(String username, String password, HttpSession session, HttpServletRequest request, HttpServletResponse response) { return service.doLogin(username.trim(), password.trim(), session, request, response).toJSONString(); }
業(yè)務(wù)層
/** * 執(zhí)行登錄 */public JSONObject doLogin(String username, String password, HttpSession session, HttpServletRequest request, HttpServletResponse response) {// 最終返回的對象 JSONObject res = new JSONObject(); res.put('code', 0); if (StringUtils.isEmpty(username) || StringUtils.isEmpty(password)) { res.put('msg', '請輸入手機(jī)號或密碼'); return res; } UserInfo dbUser = dao.getUserInfoByAccount(username); if (null == dbUser) { res.put('msg', '該賬號不存在,請檢查后重試'); return res; } // 驗(yàn)證密碼是否正確 String newPassword = PasswordUtils.getMd5(password, username, dbUser.getSalt()); if (!newPassword.equals(dbUser.getPassword())) { res.put('msg', '手機(jī)號或密碼錯誤,請檢查后重試'); return res; } // 判斷賬戶狀態(tài) if (1 != dbUser.getStatus()) { res.put('msg', '該賬號已被凍結(jié),請聯(lián)系管理員'); return res; } // 將登錄用戶信息保存到session中 session.setAttribute(Const.SYSTEM_USER_SESSION, dbUser); // 保存cookie,實(shí)現(xiàn)自動登錄 Cookie cookie_username = new Cookie('cookie_username', username); // 設(shè)置cookie的持久化時間,30天 cookie_username.setMaxAge(30 * 24 * 60 * 60); // 設(shè)置為當(dāng)前項(xiàng)目下都攜帶這個cookie cookie_username.setPath(request.getContextPath()); // 向客戶端發(fā)送cookie response.addCookie(cookie_username); res.put('code', 1); res.put('msg', '登錄成功'); return res;}
4、注銷登錄
/** * 退出登錄 */@RequestMapping(value = 'logout')public String logout(HttpSession session, HttpServletRequest request, HttpServletResponse response) { // 刪除session里面的用戶信息 session.removeAttribute(Const.SYSTEM_USER_SESSION); // 保存cookie,實(shí)現(xiàn)自動登錄 Cookie cookie_username = new Cookie('cookie_username', ''); // 設(shè)置cookie的持久化時間,0 cookie_username.setMaxAge(0); // 設(shè)置為當(dāng)前項(xiàng)目下都攜帶這個cookie cookie_username.setPath(request.getContextPath()); // 向客戶端發(fā)送cookie response.addCookie(cookie_username); return 'login';}
注銷登錄時,我們需要刪除 session 里面的用戶信息,刪除 cookie 里面的用戶信息,然后請求到登錄界面
四、總結(jié)
以上就是 SpringBoot 中使用 Cookie 實(shí)現(xiàn)記住登錄功能,在項(xiàng)目中還算是比較實(shí)用的功能,希望能對正在閱讀的你一點(diǎn)點(diǎn)幫助和啟發(fā),更多相關(guān)SpringBoot Cookie記住登錄內(nèi)容請搜索好吧啦網(wǎng)以前的文章或繼續(xù)瀏覽下面的相關(guān)文章希望大家以后多多支持好吧啦網(wǎng)!
相關(guān)文章:
1. html中的form不提交(排除)某些input 原創(chuàng)2. ASP常用日期格式化函數(shù) FormatDate()3. 開發(fā)效率翻倍的Web API使用技巧4. XMLHTTP資料5. CSS3實(shí)現(xiàn)動態(tài)翻牌效果 仿百度貼吧3D翻牌一次動畫特效6. asp.net core項(xiàng)目授權(quán)流程詳解7. vue使用moment如何將時間戳轉(zhuǎn)為標(biāo)準(zhǔn)日期時間格式8. CSS3中Transition屬性詳解以及示例分享9. jsp文件下載功能實(shí)現(xiàn)代碼10. ASP動態(tài)網(wǎng)頁制作技術(shù)經(jīng)驗(yàn)分享
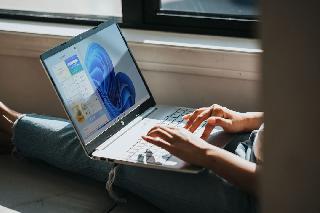