SpringBoot中自定義注解實現參數非空校驗的示例
前言
由于剛寫項目不久,在寫 web 后臺接口時,經常會對前端傳入的參數進行一些規則校驗,如果入參較少還好,一旦需要校驗的參數比較多,那么使用 if 校驗會帶來大量的重復性工作,并且代碼看起來會非常冗余,所以我首先想到能否通過一些手段改進這點,讓 Controller 層減少參數校驗的冗余代碼,提升代碼的可閱讀性。
經過閱讀他人的代碼,發現使用 annotation 注解是一個比較方便的手段,SpringBoot 自帶的 @RequestParam 注解只會校驗請求中該參數是否存在,但是該參數是否符合一些規格比如不為 null 且不為空就無法進行判斷的,所以我們可以嘗試一下增強請求參數中的注解。
準備工作
有了前面的思路,我們先搭一個架子出來。
SpringBoot 2.3.5.REALEASE JDK 1.8pom.xml 文件如下:
<?xml version='1.0' encoding='UTF-8'?><project xmlns='http://maven.apache.org/POM/4.0.0' xmlns:xsi='http://www.w3.org/2001/XMLSchema-instance' xsi:schemaLocation='http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd'> <modelVersion>4.0.0</modelVersion> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.3.5.RELEASE</version> <relativePath/> <!-- lookup parent from repository --> </parent> <groupId>cn.bestzuo</groupId> <artifactId>springboot-annotation</artifactId> <version>0.0.1-SNAPSHOT</version> <name>springboot-annotation</name> <description>Demo project for Spring Boot</description> <properties> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-thymeleaf</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.projectlombok</groupId> <artifactId>lombok</artifactId> <optional>true</optional> </dependency> <!--引入AOP相應的注解--> <dependency> <groupId>org.aspectj</groupId> <artifactId>aspectjweaver</artifactId> <version>1.8.5</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> <exclusions> <exclusion> <groupId>org.junit.vintage</groupId> <artifactId>junit-vintage-engine</artifactId> </exclusion> </exclusions> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build></project>
其中 aspectjweaver 用于引入 AOP 的相關的注解,如 @Aspect、@Pointcut 等.
使用自定義注解實現統一非空校驗
總體思路:自定義一個注解,對必填的參數加上該注解,然后定義一個切面,校驗該參數是否為空,如果為空則拋出自定義的異常,該異常被自定義的異常處理器捕獲,然后返回相應的錯誤信息。
1.自定義注解
創建一個名為 ParamCheck 的注解,代碼如下:
package cn.bestzuo.springbootannotation.annotation;import java.lang.annotation.ElementType;import java.lang.annotation.Retention;import java.lang.annotation.RetentionPolicy;import java.lang.annotation.Target;/** * 參數不能為空注解,作用于方法參數上 * * @author zuoxiang * @since 2020-11-11 */@Target(ElementType.PARAMETER)@Retention(RetentionPolicy.RUNTIME)public @interface ParamCheck { /** * 是否非空,默認不能為空 */ boolean notNull() default true;}
其中 @Target 注解中的 ElementType.PARAMETER 表示該注解的作用范圍,我們查看源碼可以看到,注解的作用范圍定義比較廣泛,可以作用于方法、參數、構造方法、本地變量、枚舉等等。
public enum ElementType { /** Class, interface (including annotation type), or enum declaration */ TYPE, /** Field declaration (includes enum constants) */ FIELD, /** Method declaration */ METHOD, /** Formal parameter declaration */ PARAMETER, /** Constructor declaration */ CONSTRUCTOR, /** Local variable declaration */ LOCAL_VARIABLE, /** Annotation type declaration */ ANNOTATION_TYPE, /** Package declaration */ PACKAGE, /** * Type parameter declaration * * @since 1.8 */ TYPE_PARAMETER, /** * Use of a type * * @since 1.8 */ TYPE_USE}
當然,我們定義的注解可以擴展,不僅僅去校驗參數是否為空,比如我們可以增加字符串長度的校驗。
2.自定義異常類
我們在這里自定義異常的原因,是為了配合自定義注解使用,一旦校驗出不符合我們自定義注解規格的參數,可以直接拋出自定義異常返回。代碼如下:
package cn.bestzuo.springbootannotation.exception;public class ParamIsNullException extends RuntimeException { private final String parameterName; private final String parameterType; public ParamIsNullException(String parameterName, String parameterType) { super(''); this.parameterName = parameterName; this.parameterType = parameterType; } /** * 重寫了該方法 * * @return 異常消息通知 */ @Override public String getMessage() { return 'Required ' + this.parameterType + ' parameter ’' + this.parameterName + '’ must be not null !'; } public final String getParameterName() { return this.parameterName; } public final String getParameterType() { return this.parameterType; }}
該異常繼承 RuntimeException,并定義了兩個成員屬性、重寫了 getMessage() 方法之所以自定義該異常,而不用現有的 org.springframework.web.bind.MissingServletRequestParameterException 類,是因為 MissingServletRequestParameterException為Checked 異常,在動態代理過程中,很容易引發 java.lang.reflect.UndeclaredThrowableException 異常。
3.自定義 AOP
代碼如下:
package cn.bestzuo.springbootannotation.aop;import cn.bestzuo.springbootannotation.annotation.ParamCheck;import cn.bestzuo.springbootannotation.exception.ParamIsNullException;import org.aspectj.lang.JoinPoint;import org.aspectj.lang.ProceedingJoinPoint;import org.aspectj.lang.annotation.*;import org.aspectj.lang.reflect.MethodSignature;import org.slf4j.Logger;import org.slf4j.LoggerFactory;import org.springframework.stereotype.Component;import java.lang.annotation.Annotation;import java.lang.reflect.Method;@Component@Aspectpublic class ParamCheckAop { private static final Logger LOGGER = LoggerFactory.getLogger(ParamCheckAop.class); /** * 定義有一個切入點,范圍為 controller 包下的類 */ @Pointcut('execution(public * cn.bestzuo.controller..*.*(..))') public void checkParam() { } @Before('checkParam()') public void doBefore(JoinPoint joinPoint) { } /** * 檢查參數是否為空 * * @param pjp 連接點 * @return 對象 * @throws Throwable 異常 */ @Around('checkParam()') public Object doAround(ProceedingJoinPoint pjp) throws Throwable { MethodSignature signature = ((MethodSignature) pjp.getSignature()); //得到攔截的方法 Method method = signature.getMethod(); //獲取方法參數注解,返回二維數組是因為某些參數可能存在多個注解 Annotation[][] parameterAnnotations = method.getParameterAnnotations(); if (parameterAnnotations.length == 0) { return pjp.proceed(); } //獲取方法參數名 String[] paramNames = signature.getParameterNames(); //獲取參數值 Object[] paramValues = pjp.getArgs(); //獲取方法參數類型 Class<?>[] parameterTypes = method.getParameterTypes(); for (int i = 0; i < parameterAnnotations.length; i++) { for (int j = 0; j < parameterAnnotations[i].length; j++) { //如果該參數前面的注解是ParamCheck的實例,并且notNull()=true,則進行非空校驗 if (parameterAnnotations[i][j] != null && parameterAnnotations[i][j] instanceof ParamCheck && ((ParamCheck) parameterAnnotations[i][j]).notNull()) { paramIsNull(paramNames[i], paramValues[i], parameterTypes[i] == null ? null : parameterTypes[i].getName()); break; } } } return pjp.proceed(); } /** * 在切入點return內容之后切入內容(可以用來對處理返回值做一些加工處理) * * @param joinPoint 連接點 */ @AfterReturning('checkParam()') public void doAfterReturning(JoinPoint joinPoint) { } /** * 參數非空校驗,如果參數為空,則拋出ParamIsNullException異常 * * @param paramName 參數名稱 * @param value 參數值 * @param parameterType 參數類型 */ private void paramIsNull(String paramName, Object value, String parameterType) { if (value == null || ''.equals(value.toString().trim())) { throw new ParamIsNullException(paramName, parameterType); } }}
4.全局異常處理器
該異常處理器捕獲在 ParamCheckAop 類中拋出的 ParamIsNullException 異常,并進行處理,代碼如下:
import cn.bestzuo.springbootannotation.common.Result;import cn.bestzuo.springbootannotation.enums.EnumResultCode;import cn.bestzuo.springbootannotation.utils.ResponseMsgUtil;import org.slf4j.Logger;import org.slf4j.LoggerFactory;import org.springframework.web.bind.MissingServletRequestParameterException;import org.springframework.web.bind.annotation.ExceptionHandler;import javax.servlet.http.HttpServletRequest;public class GlobalExceptionHandler { private static final Logger LOGGER = LoggerFactory.getLogger(GlobalExceptionHandler.class); /** * 參數為空異常處理 * * @param ex 異常 * @return 返回的異常 */ @ExceptionHandler({MissingServletRequestParameterException.class, ParamIsNullException.class}) public Result<String> requestMissingServletRequest(Exception ex) { LOGGER.error('request Exception:', ex); return ResponseMsgUtil.builderResponse(EnumResultCode.FAIL.getCode(), ex.getMessage(), null); } /** * 特別說明: 可以配置指定的異常處理,這里處理所有 * * @param request 請求 * @param e 異常體 * @return 返回的異常 */ @ExceptionHandler(value = Exception.class) public Result<String> errorHandler(HttpServletRequest request, Exception e) { LOGGER.error('request Exception:', e); return ResponseMsgUtil.exception(); }}
5.測試
首先定義一個 Controller 進行測試:
@RestControllerpublic class HelloController { /** * 測試@RequestParam注解 * * @param name 測試參數 * @return 包裝結果 */ @GetMapping('/hello1') public Result<String> hello1(@RequestParam String name) { return ResponseMsgUtil.builderResponse(EnumResultCode.SUCCESS.getCode(), '請求成功', 'Hello,' + name); } /** * 測試@ParamCheck注解 * * @param name 測試參數 * @return 包裝結果 */ @GetMapping('/hello2') public Result<String> hello2(@ParamCheck String name) { return ResponseMsgUtil.builderResponse(EnumResultCode.SUCCESS.getCode(), '請求成功', 'Hello,' + name); } /** * 測試@ParamCheck與@RequestParam一起時 * * @param name 測試參數 * @return 包裝結果 */ @GetMapping('/hello3') public Result<String> hello3(@ParamCheck @RequestParam String name) { return ResponseMsgUtil.builderResponse(EnumResultCode.SUCCESS.getCode(), '請求成功', 'Hello,' + name); }}
測試訪問 http://localhost:8080/hello1,此時只有 @RequestParam 注解,如果不加 name 參數,會請求得到一個異常:
并且控制臺會報 MissingServletRequestParameterException: Required String parameter ’name’ is not present] 異常
如果訪問 http://localhost:8080/hello2?name=,此時使用的是我們自定義的 @ParamCheck 注解,此時沒有參數輸入,那么也會捕獲輸入的異常:
如果訪問 http://localhost:8080/hello3?name=,此時既有參數存在校驗,又有我們自定義的 ParamCheck 不為空校驗,所以此時訪問不加參數會拋出異常:
控制臺拋出我們自定義的異常:
測試總結:
當參數名為空時,分別添加兩個注解的接口都會提示參數不能為空當參數名不為空,值為空時,@RequestParam注解不會報錯,但@ParamCheck注解提示參數’name’的值為空
6.總結
經過以上的測試也驗證了 @RequestParam 只會驗證對應的參數是否存在,而不會驗證值是否為空 ParamCheck 還可以進行拓展,比如參數值長度、是否含有非法字符等校驗7.代碼附錄
上述使用到的代碼:
package cn.bestzuo.springbootannotation.common;import lombok.Getter;import lombok.Setter;@Getter@Setterpublic class Result<T> { private Integer resCode; private String resMsg; private T data;}
package cn.bestzuo.springbootannotation.enums;/** * 枚舉參數結果 * * @author zuoxiang * @since 2020-11-11 */public enum EnumResultCode { SUCCESS(200), FAIL(400), UNAUTHORIZED(401), NOT_FOUND(404), INTERNAL_SERVER_ERROR(500); private final int code; EnumResultCode(int code) { this.code = code; } public int getCode() { return code; }}
package cn.bestzuo.springbootannotation.utils;import cn.bestzuo.springbootannotation.common.Result;import cn.bestzuo.springbootannotation.enums.EnumResultCode;public class ResponseMsgUtil { /** * 根據消息碼等生成接口返回對象 * * @param code 結果返回碼 * @param msg 結果返回消息 * @param data 數據對象 * @param <T> 泛型 * @return 包裝對象 */ public static <T> Result<T> builderResponse(int code, String msg, T data) { Result<T> res = new Result<>(); res.setResCode(code); res.setResMsg(msg); res.setData(data); return res; } /** * 請求異常返回結果 * * @param <T> 泛型 * @return 包裝對象 */ public static <T> Result<T> exception() { return builderResponse(EnumResultCode.INTERNAL_SERVER_ERROR.getCode(), '服務異常', null); }}
以上就是SpringBoot中自定義注解實現參數非空校驗的示例的詳細內容,更多關于SpringBoot 參數非空校驗的資料請關注好吧啦網其它相關文章!
相關文章:
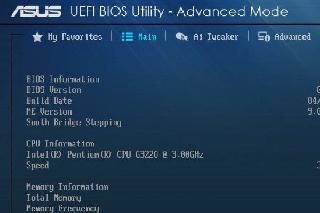