java多線程CountDownLatch與線程池ThreadPoolExecutor/ExecutorService案例
一個同步工具類,它允許一個或多個線程一直等待,直到其他線程的操作執(zhí)行完后再執(zhí)行。
2、ThreadPoolExecutor/ExecutorService:線程池,使用線程池可以復(fù)用線程,降低頻繁創(chuàng)建線程造成的性能消耗,同時對線程的創(chuàng)建、啟動、停止、銷毀等操作更簡便。
3、使用場景舉例:年末公司組織團建,要求每一位員工周六上午8點到公司門口集合,統(tǒng)一乘坐公司所租大巴前往目的地。
在這個案例中,公司作為主線程,員工作為子線程。
4、代碼示例:package com.test.thread;import java.util.concurrent.CountDownLatch;import java.util.concurrent.ExecutorService;import java.util.concurrent.Executors;import java.util.concurrent.LinkedBlockingQueue;import java.util.concurrent.ThreadPoolExecutor;import java.util.concurrent.TimeUnit;/** * @author javaloveiphone * @date 創(chuàng)建時間:2017年1月25日 上午10:59:11 * @Description: */public class Company { public static void main(String[] args) throws InterruptedException { //員工數(shù)量 int count = 5; //創(chuàng)建計數(shù)器 //構(gòu)造參數(shù)傳入的數(shù)量值代表的是latch.countDown()調(diào)用的次數(shù) CountDownLatch latch = new CountDownLatch(count); //創(chuàng)建線程池,可以通過以下方式創(chuàng)建 //ThreadPoolExecutor threadPool = new ThreadPoolExecutor(1,1,60,TimeUnit.SECONDS,new LinkedBlockingQueue<Runnable>(count)); ExecutorService threadPool = Executors.newFixedThreadPool(count); System.out.println('公司發(fā)送通知,每一位員工在周六早上8點到公司大門口集合'); for(int i =0;i<count ;i++){ //將子線程添加進線程池執(zhí)行 Thread.sleep(10); threadPool.execute(new Employee(latch,i+1)); } try { //阻塞當(dāng)前線程,直到所有員工到達公司大門口之后才執(zhí)行 latch.await(); // 使當(dāng)前線程在鎖存器倒計數(shù)至零之前一直等待,除非線程被中斷或超出了指定的等待時間。 //latch.await(long timeout, TimeUnit unit) System.out.println('所有員工已經(jīng)到達公司大門口,大巴車發(fā)動,前往活動目的地。'); } catch (InterruptedException e) { e.printStackTrace(); }finally{ //最后關(guān)閉線程池,但執(zhí)行以前提交的任務(wù),不接受新任務(wù) threadPool.shutdown(); //關(guān)閉線程池,停止所有正在執(zhí)行的活動任務(wù),暫停處理正在等待的任務(wù),并返回等待執(zhí)行的任務(wù)列表。 //threadPool.shutdownNow(); } }}//分布式工作線程class Employee implements Runnable{ private CountDownLatch latch; private int employeeIndex; public Employee(CountDownLatch latch,int employeeIndex){ this.latch = latch; this.employeeIndex = employeeIndex; } @Override public void run() { try { System.out.println('員工:'+employeeIndex+',正在前往公司大門口集合...'); Thread.sleep(10); System.out.println('員工:'+employeeIndex+',已到達。'); } catch (Exception e) { e.printStackTrace(); }finally{ //當(dāng)前計算工作已結(jié)束,計數(shù)器減一 latch.countDown(); try { Thread.sleep(10); } catch (InterruptedException e) { e.printStackTrace(); } //執(zhí)行coutDown()之后,繼續(xù)執(zhí)行自己的工作,不受主線程的影響 System.out.println('員工:'+employeeIndex+',吃飯、喝水、拍照。'); } }}
打印輸出結(jié)果如下:
公司發(fā)送通知,每一位員工在周六早上8點到公司大門口集合員工:1,正在前往公司大門口集合...員工:1,已到達。員工:2,正在前往公司大門口集合...員工:2,已到達。員工:1,吃飯、喝水、拍照。員工:3,正在前往公司大門口集合...員工:2,吃飯、喝水、拍照。員工:3,已到達。員工:4,正在前往公司大門口集合...員工:3,吃飯、喝水、拍照。員工:4,已到達。員工:5,正在前往公司大門口集合...員工:4,吃飯、喝水、拍照。員工:5,已到達。所有員工已經(jīng)到達公司大門口,大巴車發(fā)動,前往活動目的地。員工:5,吃飯、喝水、拍照。注意:
每一個員工到達之后,執(zhí)行countDown()方法,直到所有員工到達之后,計數(shù)器為0,主線程才會繼續(xù)執(zhí)行。
但子線程執(zhí)行了countDown()方法,之后會繼續(xù)自己的工作,比如上面的【吃飯、喝水、拍照】,是不受主線程是否阻塞、其它線程是否已經(jīng)執(zhí)行countDown()方法的影響的。
5、CountDownLatch與CyclicBarrier的對比可以看:java多線程CyclicBarrier使用示例,讓線程起步走
補充:CountDownLatch踩過的坑
線上生產(chǎn)環(huán)境dubbo報線程池滿了,經(jīng)過一天排查鎖定在開三個線程計算最后合并數(shù)據(jù)的步驟中。簡單描述下該步驟線程開三個 調(diào)用三個不同的方法 使用countdownlatch 計數(shù)器等待三個方法全部執(zhí)行完成 合并數(shù)據(jù)。
但是由于其中一個方法調(diào)用第三方接口,接口返回異常導(dǎo)致轉(zhuǎn)換數(shù)據(jù)報錯。導(dǎo)致其中一個方法未正常完成。
舉例demo:
public static void main(String[] args) { ExecutorService executorService =Executors.newFixedThreadPool(3); CountDownLatch cdl = new CountDownLatch(3); executorService.execute(new Runnable() { @Override public void run() { /*try { function1(); } catch (Exception e) { //異常處理 e.printStackTrace(); } finally { cdl.countDown(); }*/ function1(); } }); executorService.execute(new Runnable() { @Override public void run() { function2(); cdl.countDown(); } }); executorService.execute(new Runnable() { @Override public void run() { function3(); cdl.countDown(); } }); try { cdl.await(); //cdl.await(20,TimeUnit.SECONDS); System.out.println('三個執(zhí)行線程結(jié)束'); } catch (InterruptedException e) { e.printStackTrace(); System.out.println('執(zhí)行線程異常'); } finally { executorService.shutdown(); System.out.println('執(zhí)行線程關(guān)閉'); }}private static void function1(){ int i = 10/0; System.out.println('方法一');}private static void function2(){ System.out.println('方法二');}private static void function3(){ System.out.println('方法三');}
方法一拋出異常,但是沒有做異常處理導(dǎo)致不會執(zhí)行線程關(guān)閉步驟,是不是和想象中不一樣,一開始我也是懵,看了一下CountDownLatch原理就很好理解了,
“CountDownLatch是通過一個計數(shù)器來實現(xiàn)的,計數(shù)器的初始化值為線程的數(shù)量。每當(dāng)一個線程完成了自己的任務(wù)后,計數(shù)器的值就相應(yīng)得減1。當(dāng)計數(shù)器到達0時,表示所有的線程都已完成任務(wù),然后在閉鎖上等待的線程就可以恢復(fù)執(zhí)行任務(wù)。”【1】
舉一個現(xiàn)實中例子就是:CountDownLatch 就像跑步比賽中的裁判,三個方法就是就是三位運動員,運動員2,3都已經(jīng)到達終點,但是運動員1摔倒了,動不了。裁判員只看到兩位運動員到達終點不能宣布比賽結(jié)束,所以一直等。。。
就像這樣的場景導(dǎo)致線上service執(zhí)行線程阻塞,接口調(diào)用次數(shù)累計導(dǎo)致dubbo線程滿了(跟dubbo線程模型有關(guān),有時間具體談?wù)勥@一點)
知道原因了,就要考慮怎么修改比賽不能無限期等,所以比賽必須在有限時間內(nèi)結(jié)束,所以使用
public boolean await(long timeout, TimeUnit unit) throws InterruptedException { return sync.tryAcquireSharedNanos(1, unit.toNanos(timeout));}
線程內(nèi)部也許要增加異常處理
executorService.execute(new Runnable() { @Override public void run() { try { function1(); } catch (Exception e) { //異常處理 e.printStackTrace(); } finally { cdl.countDown(); } // function1(); }});修改后demo
public static void main(String[] args) { ExecutorService executorService =Executors.newFixedThreadPool(3); CountDownLatch cdl = new CountDownLatch(3); executorService.execute(new Runnable() { @Override public void run() { try { function1(); } catch (Exception e) { //異常處理 e.printStackTrace(); } finally { cdl.countDown(); } // function1(); } }); executorService.execute(new Runnable() { @Override public void run() { function2(); cdl.countDown(); } }); executorService.execute(new Runnable() { @Override public void run() { function3(); cdl.countDown(); } }); try { // cdl.await(); cdl.await(20,TimeUnit.SECONDS); System.out.println('三個執(zhí)行線程結(jié)束'); } catch (InterruptedException e) { e.printStackTrace(); System.out.println('執(zhí)行線程異常'); } finally { executorService.shutdown(); System.out.println('執(zhí)行線程關(guān)閉'); }}private static void function1(){ int i = 10/0; System.out.println('方法一');}private static void function2(){ System.out.println('方法二');}private static void function3(){ System.out.println('方法三');}
執(zhí)行結(jié)果
大家結(jié)合自己的現(xiàn)實使用修改,爬過了使用坑,記錄下分享下 ,希望能對別人有用
以上為個人經(jīng)驗,希望能給大家一個參考,也希望大家多多支持好吧啦網(wǎng)。如有錯誤或未考慮完全的地方,望不吝賜教。
相關(guān)文章:
1. vue-drag-chart 拖動/縮放圖表組件的實例代碼2. vue使用moment如何將時間戳轉(zhuǎn)為標準日期時間格式3. Android studio 解決logcat無過濾工具欄的操作4. 什么是Python變量作用域5. js select支持手動輸入功能實現(xiàn)代碼6. PHP正則表達式函數(shù)preg_replace用法實例分析7. Android Studio3.6.+ 插件搜索不到終極解決方案(圖文詳解)8. bootstrap select2 動態(tài)從后臺Ajax動態(tài)獲取數(shù)據(jù)的代碼9. Android 實現(xiàn)徹底退出自己APP 并殺掉所有相關(guān)的進程10. 一個 2 年 Android 開發(fā)者的 18 條忠告
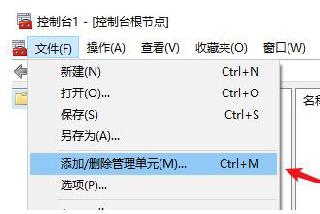