Java結束線程的三種方法及該如何選擇
java常用的結束一個運行中的線程的方法有3中:使用退出標志,使用interrupt方法,使用stop方法。
1.使用退出標志即在線程內部定義一個bool變量來判斷是否結束當前的線程:
public class ThreadSafe extends Thread { public volatile boolean exit = false; public void run() { while (!exit){ //do work } } public static void main(String[] args) throws Exception { ThreadFlag thread = new ThreadFlag(); thread.start(); sleep(5000); // 主線程延遲5秒 thread.exit = true; // 終止線程thread thread.join(); System.out.println('線程退出!'); }}
這種情況一般是將線程的任務放在run方法中的一個while循環中,而由這個bool變量來對while循環進行控制。
2.使用interrupt方法這種方法需要判斷當前的線程所處于的狀態:(1)當前線程處于阻塞狀態時線程處于阻塞狀態一般是在使用了 sleep,同步鎖的 wait,socket 中的 receiver,accept 等方法時,會使線程處于阻塞狀態。
public class ThreadInterrupt extends Thread { public void run() { try { sleep(50000); // 延遲50秒 } catch (InterruptedException e) { System.out.println(e.getMessage()); } } public static void main(String[] args) throws Exception { Thread thread = new ThreadInterrupt(); thread.start(); System.out.println('在50秒之內按任意鍵中斷線程!'); System.in.read(); thread.interrupt(); thread.join(); System.out.println('線程已經退出!'); } }
注意這種情況寫,使用 interrupt 方法結束線程的時候,一定要先捕獲 InterruptedException 異常之后通過 break 來跳出循環,才能正常結束 run 方法。
(2)線程未處于阻塞狀態時
使用 isInterrupted() 判斷線程的中斷標志來退出循環。當使用 interrupt() 方法時,中斷標志就會置 true,和使用自定義的標志來控制循環是一樣的道理。
public class ThreadSafe extends Thread { public void run() { while (!isInterrupted()) { //非阻塞過程中通過判斷中斷標志來退出 try { Thread.sleep(5*1000); //阻塞過程捕獲中斷異常來退出 } catch (InterruptedException e) { e.printStackTrace(); break; //捕獲到異常之后,執行 break 跳出循環 } } }}3.使用stop方法來結束線程
public class Main { public static void main(String[] args) throws InterruptedException { MyThread myThread = new MyThread(); myThread.start(); Thread.sleep(3000); // 間隔3秒后 myThread.stop(); // 結束線程 System.out.println('結束了'); }}4.結束方法的選擇
建議使用標志位和interrupt方法來結束線程,stop方法已經不建議再被使用了。因為采用 stop 是不安全的,主要影響點如下:
thread.stop() 調用之后,創建子線程的線程就會拋出 ThreadDeatherror 的錯誤; 調用 stop 會釋放子線程所持有的所有鎖。導致了該線程所持有的所有鎖的突然釋放(不可控制),那么被保護數據就有可能呈現不一致性。以上就是Java結束線程的三種方法及該如何選擇的詳細內容,更多關于Java 結束線程的資料請關注好吧啦網其它相關文章!
相關文章:
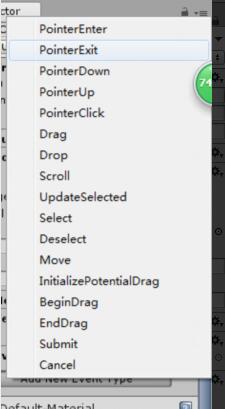