Python:__eq__和__str__函數的使用示例
一.__eq__方法
在我們定義一個類的時候,常常想對一個類所實例化出來的兩個對象進行判斷這兩個對象是否是完全相同的。一般情況下,我們認為如果同一個類實例化出來的兩個對象的屬性全都是一樣的話,那么這兩個對象是相同的。但是如果我們直接用'==”來判斷這兩個對象知否相等,那么結果一定是不相等的,因為這兩個對象的地址一定不同,它們在內存當中的不同區域,比如我們有代碼:
class Item: def __init__(self, name, weight): self.name=name self.weight=weight cat_1 = Item(’Cat’, 5)cat_2 = Item(’Cat’, 5)print(cat_1 == cat_2)
這段代碼當中,我們創建了兩個“item”對象,它們的屬性“name”和“weight”都完全一致,這段程序看似正確,應該打印出True,但實際上輸出是:
False
原因則是因為這兩個對象的地址是不同的,那么怎么才能夠讓它們只要屬性相同兩個對象就相等呢?那就是利用__eq__方法來進行判斷,這個方法默認有兩個參數,一個是self,另一個是other.也就是用自身的屬性和other對象的屬性分別進行比較,如果比對成功則返回True,失敗則返回False。你也可以自定義想要比較的屬性有哪些,也不一定是全部的屬性都一樣才相等。我們有代碼:
class Item: def __init__(self, name, weight): self.name=name self.weight=weight def __eq__(self, other): # `__eq__` is an instance method, which also accepts # one other object as an argument. if type(other)==type(self) and other.name==self.name and other.weight==self.weight: return True else: return False# 返回False這一步也是需要寫的哈,不然判斷失敗就沒有返回值了cat_1 = Item(’Cat’, 5)cat_2 = Item(’Cat’, 5)print(cat_1.__eq__(cat_2)) # should evaluate to Trueprint(cat_1 == cat_2) # should also evaluate to True
這樣,就會打印出兩個True了。
二.__str__方法
我們如果把自己創建的對象直接打印出來,那么一般是這樣,比如我們有代碼:
print(cat_1)
輸出:
<__main__.Item object at 0x7f8e3d99f190
這是一個看起來十分難看的輸出,輸出的是這對象的類別和地址。但我們可以把這個輸出改成自己想要的樣子,那就是利用__str__方法。我們重寫這個方法,讓這個返回一個值,那么最后輸出的就是我們的返回值,如下所示:
class Item: def __init__(self, name, weight): self.name=name self.weight=weight def __eq__(self, other): if type(other)==type(self) and other.name==self.name and other.weight==self.weight: return True else: return False def __str__(self): return ’the name of this cat is {}’.format(self.name)
再次創建并打印:
cat_1 = Item(’Cat’, 5)cat_2 = Item(’Cat’, 5)print(cat_1)print(cat_2)
可得到輸出:
the name of this cat is Catthe name of this cat is Cat
這樣這個輸出看起來就不會有那么麻煩了,自定義的輸出果然清晰了不少啊!
以上就是Python:__eq__和__str__函數的使用示例的詳細內容,更多關于Python __eq__和__str__函數的資料請關注好吧啦網其它相關文章!
相關文章:
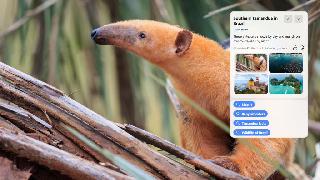